Core Java
Core Java forms the foundation of Java programming, covering essential concepts and features required to develop robust applications. This course introduces you to Java syntax, data types, control structures, and object-oriented programming (OOP) principles, including classes, objects, inheritance, and polymorphism.
Key Topics Covered:
- Java Fundamentals: Syntax, data types, variables, control statements (if, loops, switch).
- Object-Oriented Programming (OOP): Classes, objects, methods, inheritance, polymorphism, abstraction, and encapsulation.
- Advanced Java Concepts: Exception handling, multithreading, I/O handling, basic networking.
- Collections Framework: Work with Java's powerful collection classes to manage data
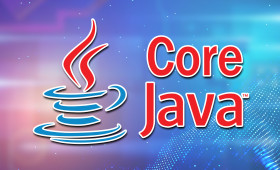
Overview
Core Java forms the foundation of Java programming, covering essential concepts and features required to develop robust applications. This course introduces you to Java syntax, data types, control structures, and object-oriented programming (OOP) principles, including classes, objects, inheritance, and polymorphism.
Key Topics Covered:
- Java Fundamentals: Syntax, data types, variables, control statements (if, loops, switch).
- Object-Oriented Programming (OOP): Classes, objects, methods, inheritance, polymorphism, abstraction, and encapsulation.
- Advanced Java Concepts: Exception handling, multithreading, I/O handling, basic networking.
- Collections Framework: Work with Java's powerful collection classes to manage data
Course Description
Introduction to Core Java
- About Java: Overview of Java programming language, its origins, and significance.
- Features: Key features of Java such as platform independence, object-oriented, multithreading, etc.
- Environment Setup: Installing Java Development Kit (JDK), setting up an IDE (like Eclipse, IntelliJ), and configuring environment variables.
- Basic "Hello World" Program: Writing your first Java program.
Basic Fundamentals of Programming
- Data Types: Primitive types (int, float, char, boolean) and reference types (objects, arrays).
- Variables: Declaration and initialization of variables, data type compatibility.
- Conditional Statements:
if
,if-else
,else-if
ladder, andnested if
for decision-making.Switch Case
: Usingswitch
for multiple conditional checks.
- Loop Statements:
For
loop: Iterating through a block of code.While
loop: Repeating code while a condition is true.Do-While
loop: Ensures at least one iteration, checks condition after execution.
Core Concepts of Java
- Class: Blueprint for creating objects; defines properties and behaviors.
- Objects: Instances of classes that hold data and methods.
- Methods:
- Functionality within a class that performs tasks.
- Method Overloading: Defining multiple methods with the same name but different parameters.
- This Keyword: Refers to the current object of the class.
- Constructors:
- Special method used for initializing objects.
- Constructor Overloading: Multiple constructors with different parameters.
Object-Oriented Programming (OOP) Concepts
-
Encapsulation: Bundling data (variables) and methods that operate on the data into a single unit (class).
- Access Modifiers:
private
,public
,protected
, and default.
- Access Modifiers:
-
Relationships:
- Has-A Relation: Composition or Aggregation.
- Is-A Relation (Inheritance): One class inherits properties and behaviors from another.
- Super Keyword: Refers to the parent class’s methods and properties.
- This vs. Super: Differences in their usage (this refers to the current class, super refers to the parent class).
-
Method Overriding: Redefining a parent class method in the child class.
-
Abstraction: Hiding implementation details and showing only essential features.
- Abstract Class: A class that cannot be instantiated but can be subclassed.
- Interfaces: Defines methods that a class must implement but without the method body.
Polymorphism
- Compile-Time Polymorphism: Also called static or early binding. Achieved through method overloading.
- Runtime Polymorphism: Also called dynamic or late binding. Achieved through method overriding.
- Overriding vs. Overloading: The key difference between method overriding and method overloading in Java.
Miscellaneous Topics
-
New, Static, Final, Instance-of Operators:
new
: Used to create objects.static
: Declaring static variables and methods (belong to the class, not instance).final
: Used for constants, methods that cannot be overridden, and classes that cannot be subclassed.instanceof
: Used to check the type of an object at runtime.
-
Package & Import Statements: Organizing code using packages and importing required classes using
import
. -
Exception Handling: Using
try
,catch
,finally
, and custom exceptions to handle runtime errors. -
Multithreading: Using
Thread
class orRunnable
interface for concurrent programming and improving performance. -
String Manipulation: String operations like concatenation, substring, length, etc., using the
String
class andStringBuilder
/StringBuffer
. -
Collections Framework:
- Interfaces like
List
,Set
, andMap
. - Implementations like
ArrayList
,HashSet
,HashMap
, etc., for storing and managing collections of objects.
- Interfaces like
This breakdown provides a structured understanding of Core Java concepts, covering both the basics and advanced features necessary for mastering Java programming.
Course Key Features
- Classroom and Online Training: Learn from the comfort of your home or in-person at our Hyderabad center.
- IT Experts as Trainers: Get trained by experienced professionals with real-world industry insights.
- Industry-Relevant Curriculum: Our course covers essential Java concepts, focusing on real-world applications.
- Hands-on Projects: Work on real-time use cases and projects to enhance practical knowledge.
- One-on-One Mentoring: Personalized attention from mentors to guide you through the learning process.
- Flexible Schedules: Choose from convenient training schedules to fit your lifestyle.
- 8 Hours of Lab Support: Daily lab sessions to practice and apply what you've learned.
- Pre-Assessment Questions: Assess your knowledge before the course begins.
- Comprehensive Course Material: Get access to well-structured study materials for self-paced learning.
- Lifetime Valid Swhizz Certification: Earn a certificate recognized for its credibility and validity.
- Resume Building: Assistance in creating a professional resume to stand out to employers.
- Interview Guidance: Receive expert tips on how to prepare for and excel in job interviews.
- Mock Interviews: Simulate real job interviews for better preparation.
- Job Drives with Top Companies: Access exclusive hiring opportunities through our extensive network.
- Internship Opportunities: Gain industry exposure through internships with leading companies.
- Tie-ups with 50+ Clients: Partnered with top firms for job placements and career support.
Join Swhizz Technologies today to kickstart your career in Java development!
Benefits
- Comprehensive Curriculum: Covers all key concepts from basics to advanced topics.
- Hands-on Learning: Apply concepts through real-time projects.
- Expert Mentors: Learn from industry experts with practical experience.
- 100% Placement Assistance: Resume building, interview preparation, and job placement support.
Enroll now to master Core Java and pave your way to a successful career in software development!
Who Should Attend
- Beginners: Individuals with no prior programming experience who want to start a career in Java development.
- Graduates: Fresh graduates seeking to build a strong foundation in Java programming for software development roles.
- Software Developers: Developers looking to enhance their skills and work with advanced Java features.
- IT Professionals: Professionals aiming to transition into Java development or improve their existing Java knowledge.
- Career Gap Candidates: Individuals returning to the workforce after a career gap and seeking to gain relevant Java development skills.
- Quality Assurance Engineers: QA professionals wanting to expand their expertise with Java for test automation and scripting.
This training is ideal for anyone interested in mastering Java programming to develop robust, scalable applications and pursue careers in Java development, software engineering, and related fields.